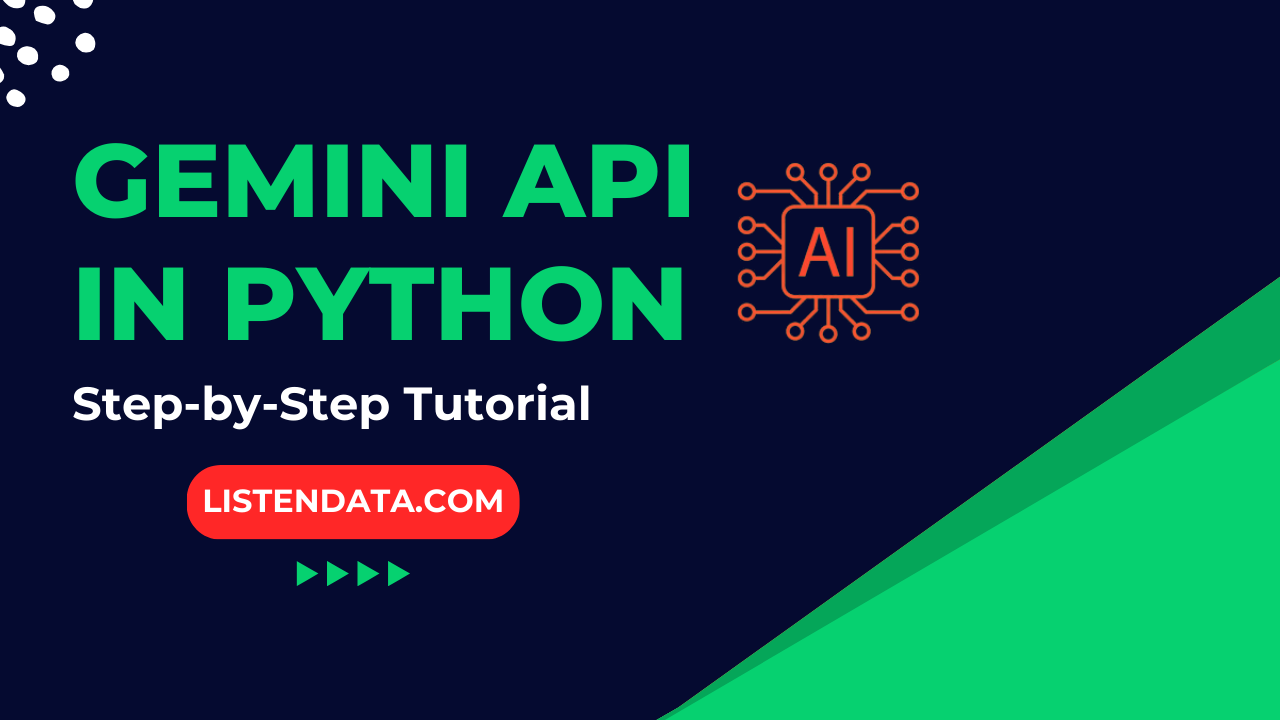
In this tutorial, you will learn how to use Google's Gemini AI model through its API in Python.
Follow the steps below to access the Gemini API and then use it in python.
- Visit Google AI Studio website.
- Sign in using your Google account.
- Create an API key.
- Install the Google AI Python library for the Gemini API using the command below :
pip install google-generativeai
.
The program below shows the easiest way to generate response of your question using generate_content() method. Please make sure to enter your API key in this section of code - ENTER_YOUR_API_KEY
.
import google.generativeai as genai
import os
os.environ["API_KEY"] = 'ENTER_YOUR_API_KEY'
genai.configure(api_key=os.environ["API_KEY"])
model = genai.GenerativeModel('gemini-1.5-pro-latest')
response = model.generate_content("President of USA is")
print(response.text)
The current President of the United States is **Joe Biden**.
You can set parameters of the model in the generation_config
argument of the 'GenerativeModel()' function.
The temperature paramater is used to control the randomness in the responses the model generates. It lies between 0 and 2. The default value for Gemini-1.5-pro is 1.0. Lower temperature means lower randomness and more predictable output. Higher temperature closer to 2 means more randomness and the responses to be more creative.
# Model Configuration
model_config = {
"temperature": 1,
"top_p": 0.99,
"top_k": 0,
"max_output_tokens": 4096,
}
model = genai.GenerativeModel('gemini-1.5-pro-latest',
generation_config=model_config)
response = model.generate_content("the most efficient way to remove duplicates of a list in python. Less verbose response.")
print(response.text)
list(set(your_list))
Often we need to converse by asking questions based on the response to the previous question. This functionality is similar to how chat works on Gemini website.
chat = model.start_chat(history=[])
response = chat.send_message("2+2")
print(response.text)
# Output
# 2 + 2 = 4
response = chat.send_message("square of it")
print(response.text)
# Output
# The square of 4 is 16
response = chat.send_message("Add 4 to it")
print(response.text)
# Output
# Adding 4 to 16 gives us 20.
You can use an image in your prompt (question). Refer the code below.
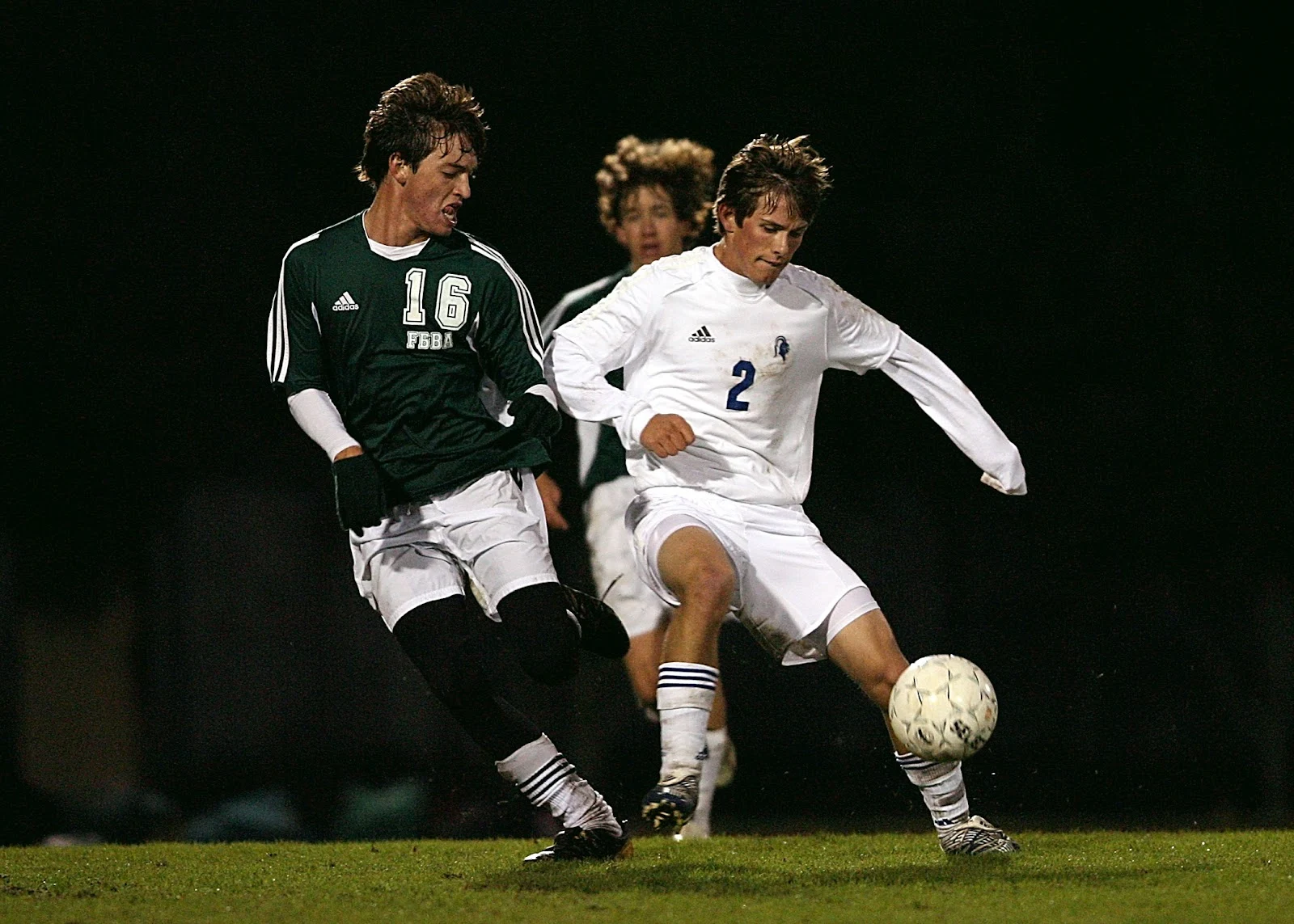
To install the libraries for handling images using pip, you can run :
pip install requests pillow
import requests from PIL import Image from io import BytesIO url = "https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEgCGchJj9jVRP0jMND1a6tJXj7RcYWtnCO4J6YcbPTXrNxiCvs_3NSk7h2gB0h2sc_6bTvwPrBeBHwUA45AXAhaw1uuINuPDcHCbARxpgJIXM5Spi_0P45aR6tqZ_yof-YlNn41LhzHjfW-wsV3mhxBug4To8xtgyMzsHLbm3XoaHZmYUdNY1YWJA5rh6cB/s1600/Soccer-1490541_960_720.jpg" response = requests.get(url) img = Image.open(BytesIO(response.content)) model = genai.GenerativeModel('models/gemini-pro-vision') prompt = """What's inside the image.""" response = model.generate_content([prompt, img]) print(response.text)
The image shows a soccer game at night. Two players, one in white and one in green, are playing the ball. The player in white is kicking the ball past the player in green.
To use images from your local device, you can specify the file path of your image directly.
img = Image.open("C:\\Users\\deepa\\Downloads\\Soccer.jpg")
By setting instructions, you can tell the AI model to always remember the important instructions while generating a response. You can set instructions in the system_instruction argument in the GenerativeModel( )
function.
# System Instruction
instruction = """You are a linguistic expert who specializes in the English language.
I will give you text to check grammar of the sentence. Provide corrected sentence."""
model = genai.GenerativeModel('gemini-1.5-pro-latest', system_instruction=instruction)
response = model.generate_content("Hello, how is you")
print(response.text)
The correct sentence is: "Hello, how are you?"
Let's say you have a large text and you want to build a chatbot where users can ask questions about the text and the chatbot will answer them.
import google.generativeai as genai
import os
os.environ["API_KEY"] = 'xxxxxxxxxxxxxxxxxxx'
genai.configure(api_key=os.environ["API_KEY"])
def make_prompt(query, passage):
escaped = passage.replace("\n", " ").replace("'", "").replace('"', "")
prompt = (
f"You are a helpful and informative bot that answers questions using text from the reference passage included below. "
f"Be sure to respond in a complete sentence, being comprehensive, including all relevant background information. "
f"However, you are talking to a non-technical audience, so be sure to break down complicated concepts and "
f"strike a friendly and conversational tone. "
f"If the passage is irrelevant to the answer, you may ignore it.\n"
f"QUESTION: '{query}'\n"
f"PASSAGE: '{escaped}'\n\n"
f"ANSWER:\n"
)
return prompt
passage = "Title: Google to show AI generated results in search result\n Author: Mr. AI\nFull article:\n It is going to impact publishers significantly. It's estimated their traffic would go down by 25%."
query = "Who is the author of this article?"
prompt = make_prompt(query, passage)
model = genai.GenerativeModel('gemini-1.5-pro-latest')
response = model.generate_content(prompt)
print(response.text)
The author of the article "Google to show AI generated results in search result" is Mr. AI.
We can generate embeddings for text to help us find the right documents when we have questions about a certain topic. For example, if we have three documents about AI, we can use the embeddings to find the most relevant document related to our question.
import google.generativeai as genai
import os
os.environ["API_KEY"] = 'xxxxxxxxxxxxxxxxxx'
genai.configure(api_key=os.environ["API_KEY"])
def embedding(prompt, model_name="models/text-embedding-004"):
response = genai.embed_content(content=prompt, model=model_name)
return response['embedding']
# Sample documents
documents = [
"AI is like a smart helper in healthcare. It can find problems early by looking at lots of information, help doctors make plans just for you, and even make new medicines faster.",
"AI needs to be open and fair. Sometimes, it can learn things that aren't right. We need to be careful and make sure it's fair for everyone. If AI makes a mistake, someone needs to take responsibility.",
"AI is making school exciting. It can make learning fit you better, help teachers make fun lessons, and show when you need more help."
]
# Embed documents and query
embeddings = embedding(documents)
query_embedding = embedding("AI can generate misleading results many times.")
# Calculate dot products
dot_products = [sum(a * b for a, b in zip(embedding, query_embedding)) for embedding in embeddings]
most_relevant_idx = dot_products.index(max(dot_products))
print(documents[most_relevant_idx])
AI needs to be open and fair. Sometimes, it can learn things that aren't right. We need to be careful and make sure it's fair for everyone. If AI makes a mistake, someone needs to take responsibility.
To see the list of latest models Gemini API supports, you can run the code below.
for model in genai.list_models():
print(model.name)
models/chat-bison-001 models/text-bison-001 models/embedding-gecko-001 models/gemini-1.0-pro models/gemini-1.0-pro-001 models/gemini-1.0-pro-latest models/gemini-1.0-pro-vision-latest models/gemini-1.5-flash-latest models/gemini-1.5-pro-latest models/gemini-pro models/gemini-pro-vision models/embedding-001 models/text-embedding-004 models/aqa
Share Share Tweet